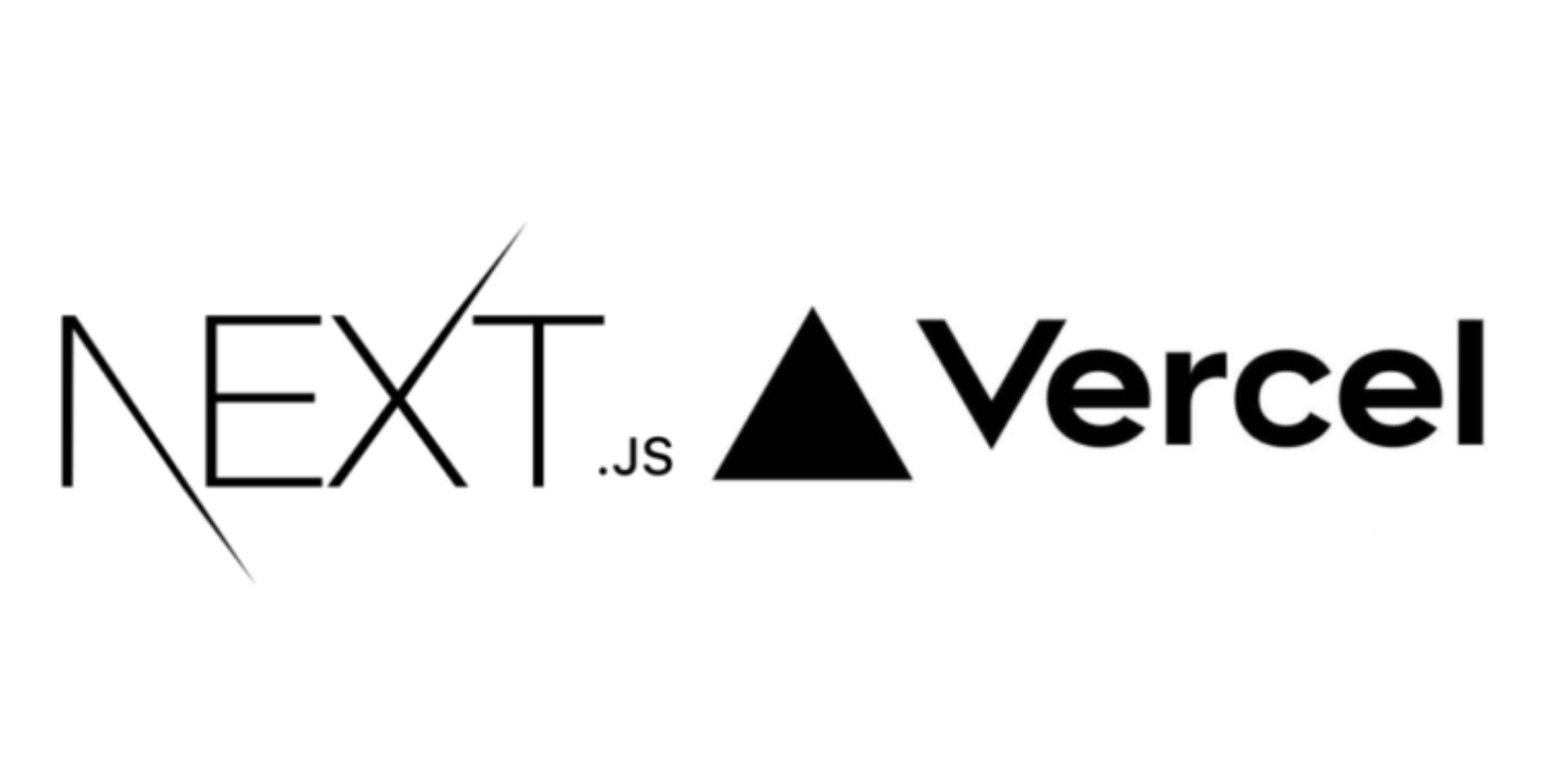
Let's take a look at how to make a blog using NextJS and VERCEL. There is also a way to make a word press blog spot, but if you make it yourself as a developer, it will be a good experience.
Create Next.js Project
npx create-next-app cozy-world
Since I'm going to make Next.js with typescript, I wrote the following.
npx create-next-app@latest --typescript cozy-world
Apply the Next.js blog template
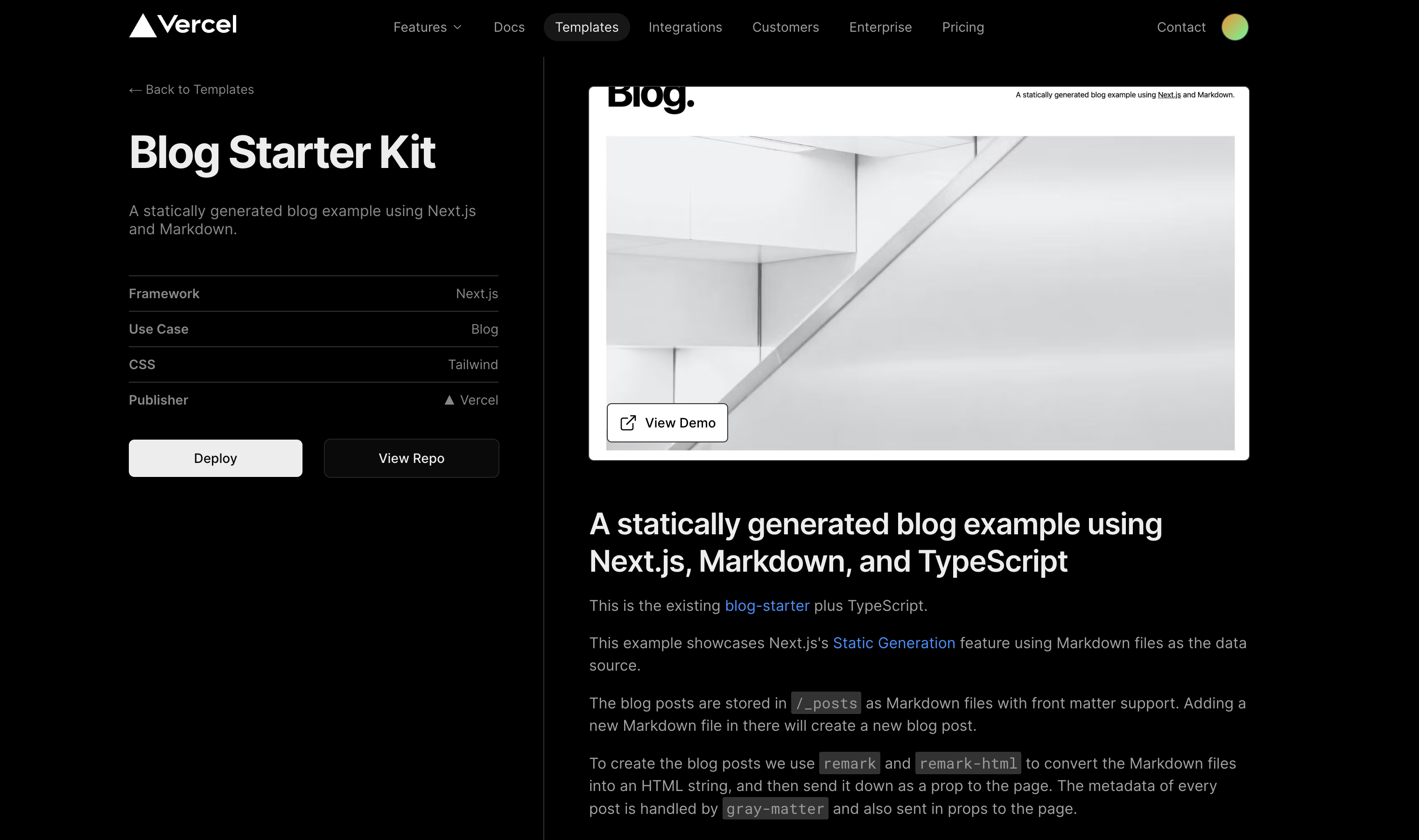
There is a blog boilerplate using Nextjs in vercel's official document, so apply it by referring to this repository.
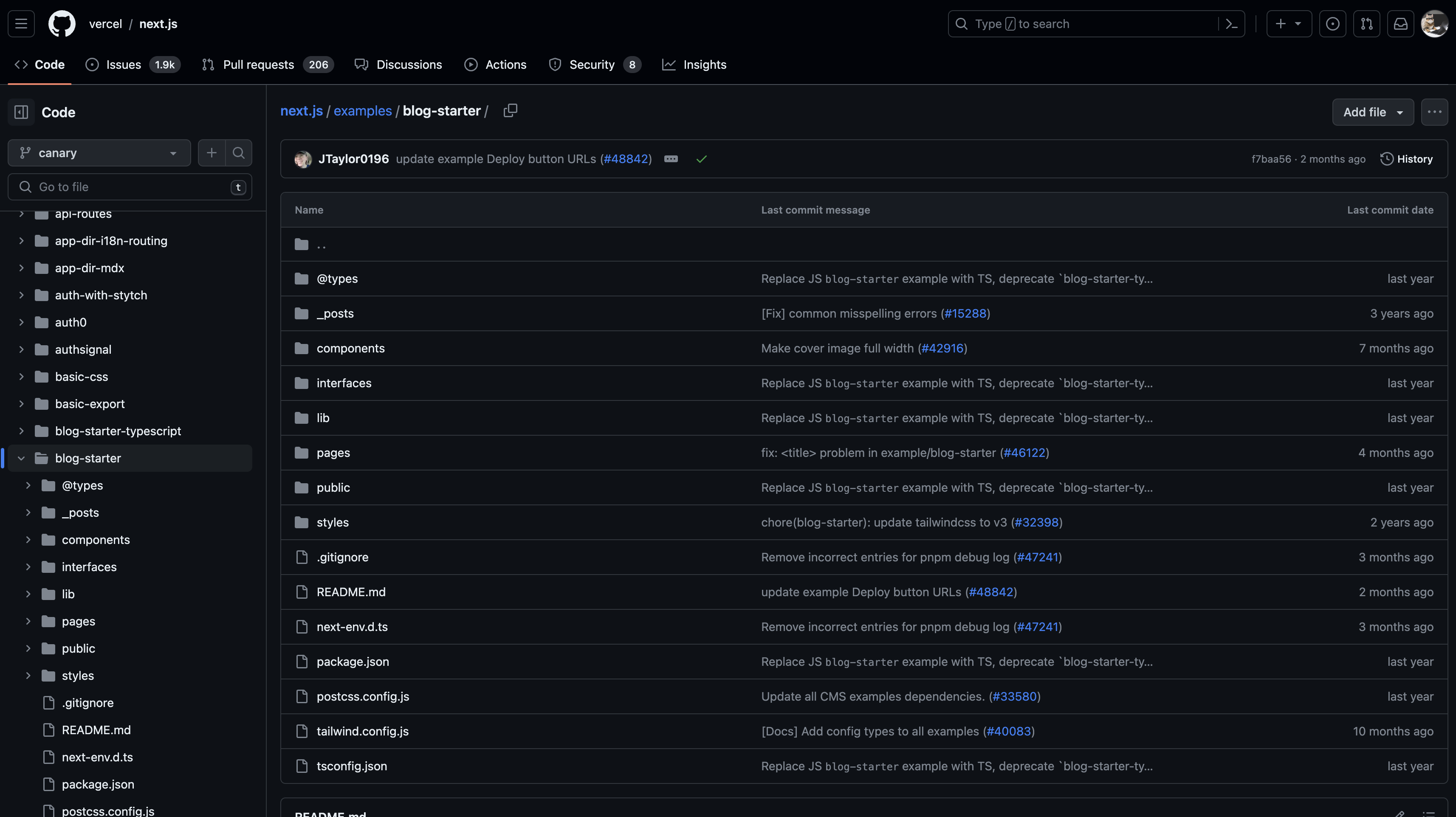
Use the blog-starter code to remove unnecessary parts and use it.
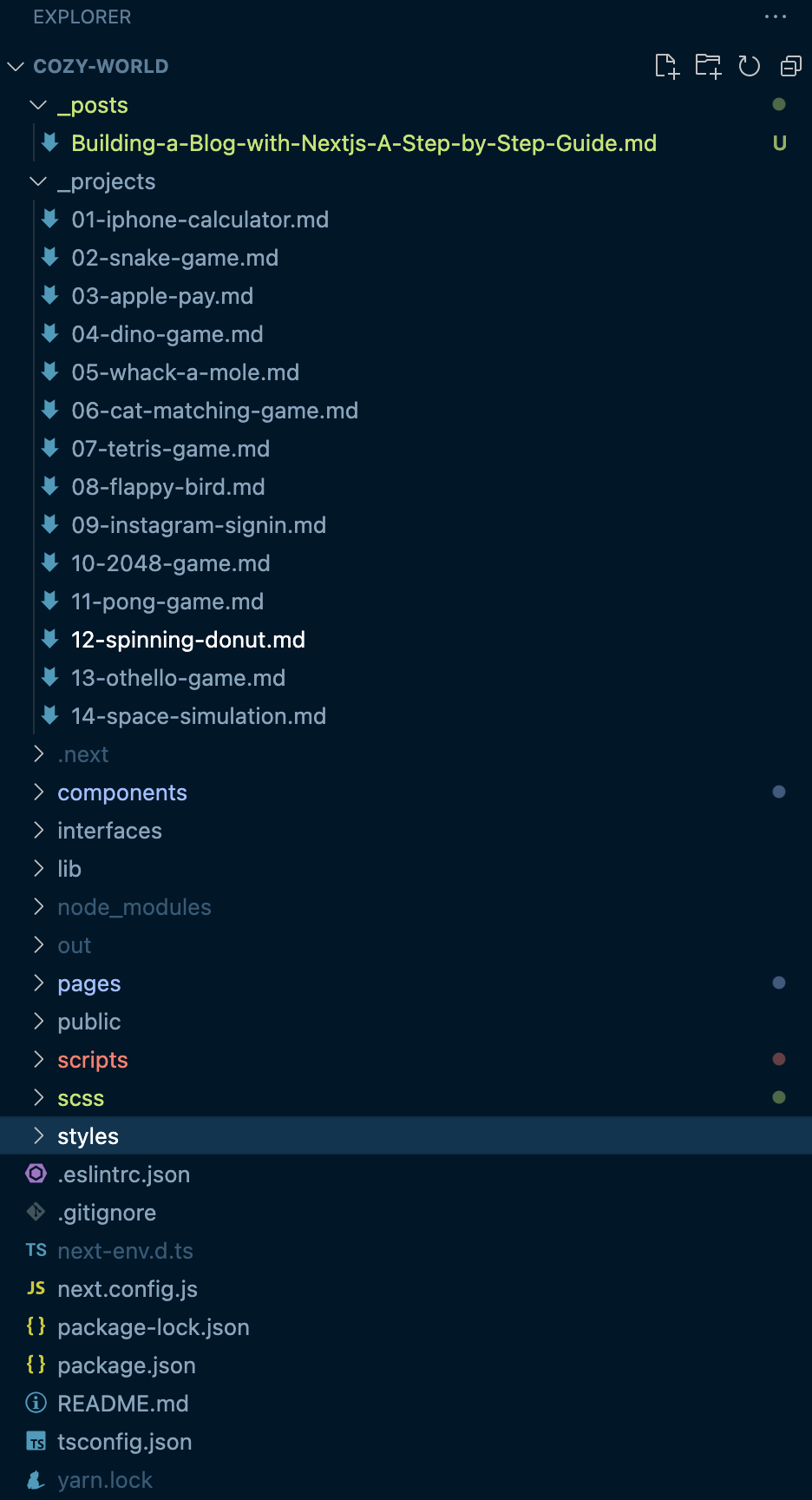
Because cozy-world is largely divided into blog posts and projects, I modified it to have the folder structure as above.
SCSS
The style of this blog uses scss.
yarn add sass
When sass is installed, you can create it by creating component.module.scss
under the component folder as shown below.
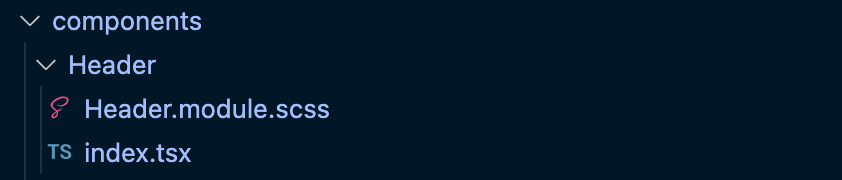
If the file name is written as module.scss, the unique class name in each component is automatically applied.
The scss file was applied using bind of classnames as shown below.
import React from "react";
import style from "./Header.module.scss";
import classnames from "classnames/bind";
import Link from "next/link";
const cx = classnames.bind(style);
const Header = () => {
return (
<header className={cx("header")}>
<div className={cx("inner")}>
<h1 className={cx("title")}>
<Link href={"/"}>Cozy World</Link>
</h1>
<nav className={cx("nav_area")}>
<Link href={"/posts"} className={cx("nav_item")}>
Posts
</Link>
<Link href={"/projects"} className={cx("nav_item")}>
Projects
</Link>
</nav>
</div>
</header>
);
};
export default Header;
If written as above, the class name is uniquely applied as shown in the picture below.
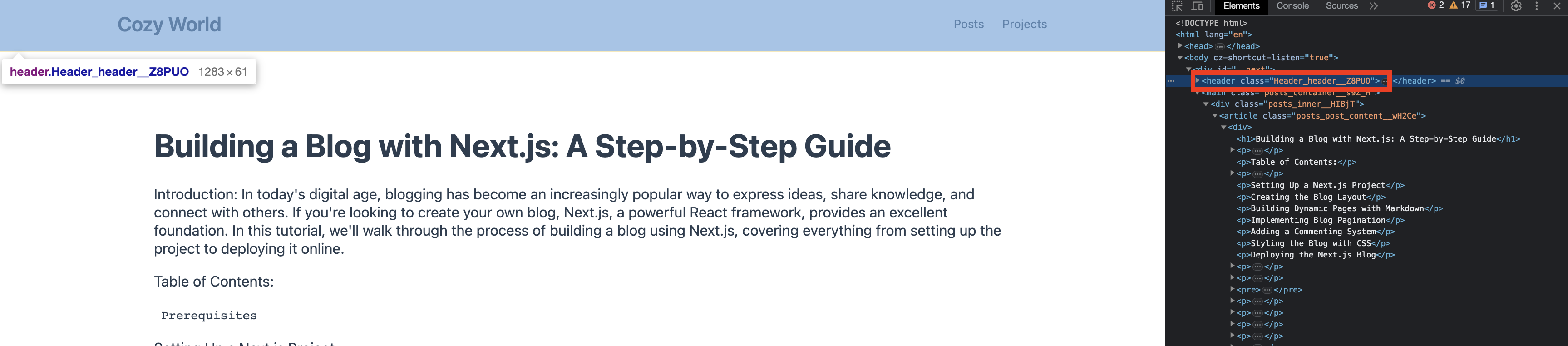
Deploy
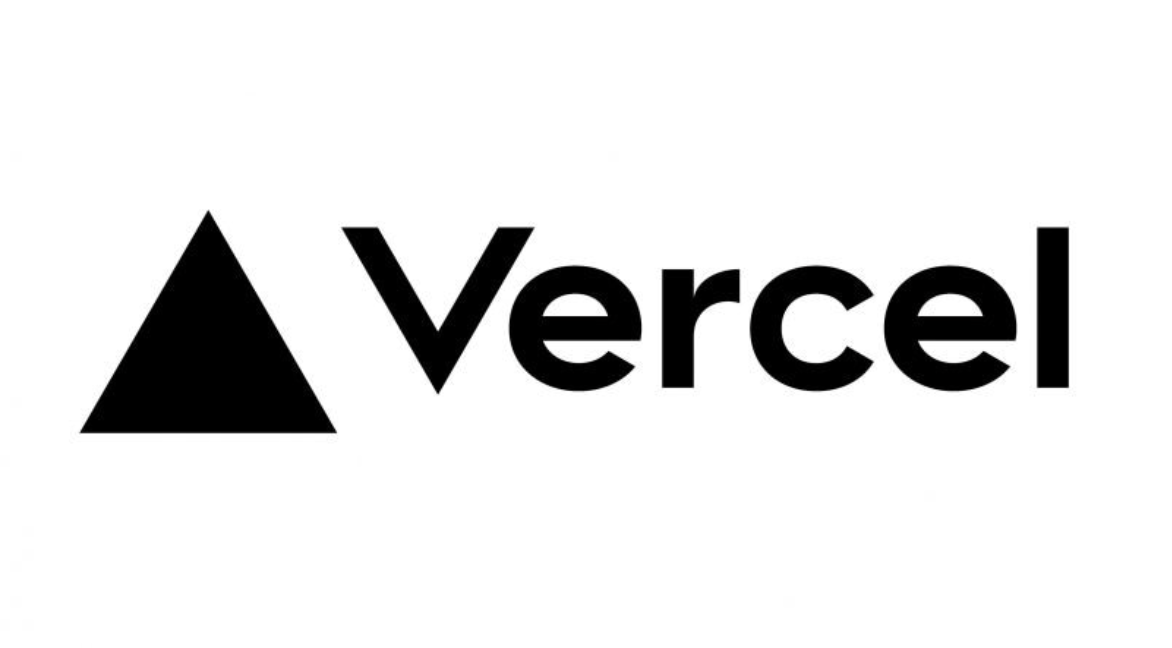
Now that we have created the basic structure for our blog and written the css, let's deploy it using vercel.
Create a new project after signing up for vercel.
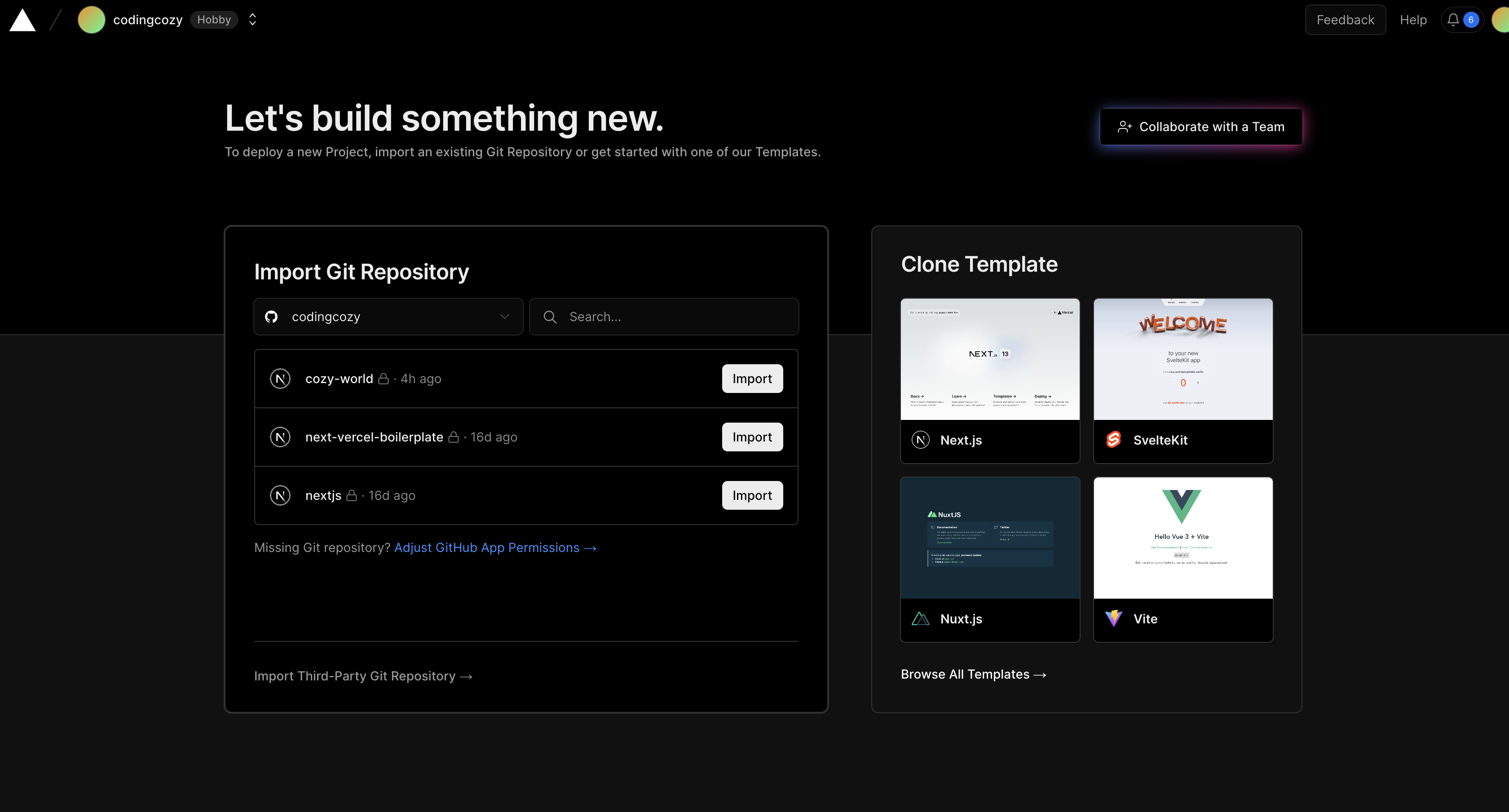
When a project is pushed to a github repository, it is automatically deployed.
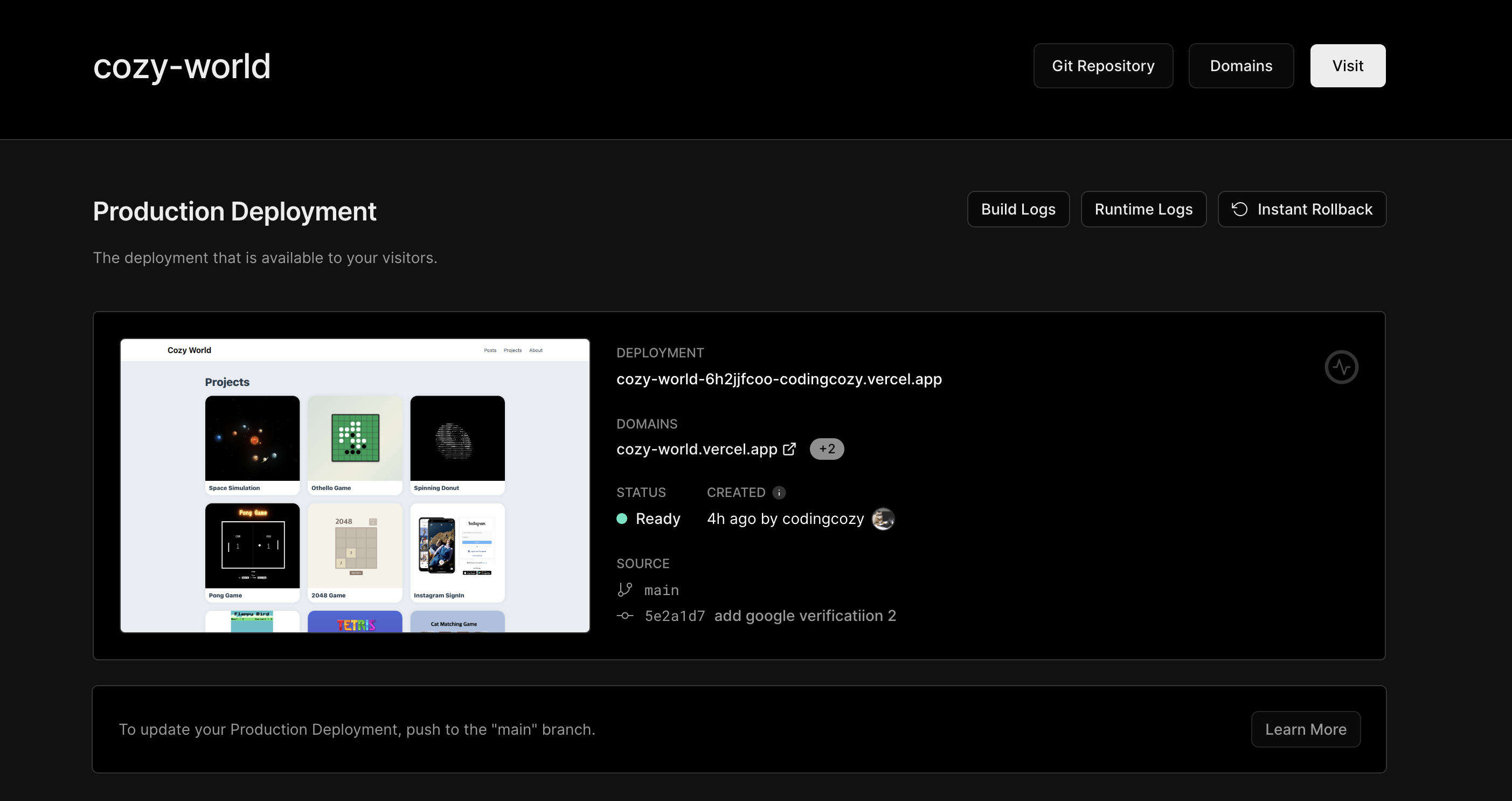
Today, I learned how to simply create a blog using Nextjs and vercel.
Next, we will cover the process of adding necessary features to the blog.